Revolutionary Enhancements in React
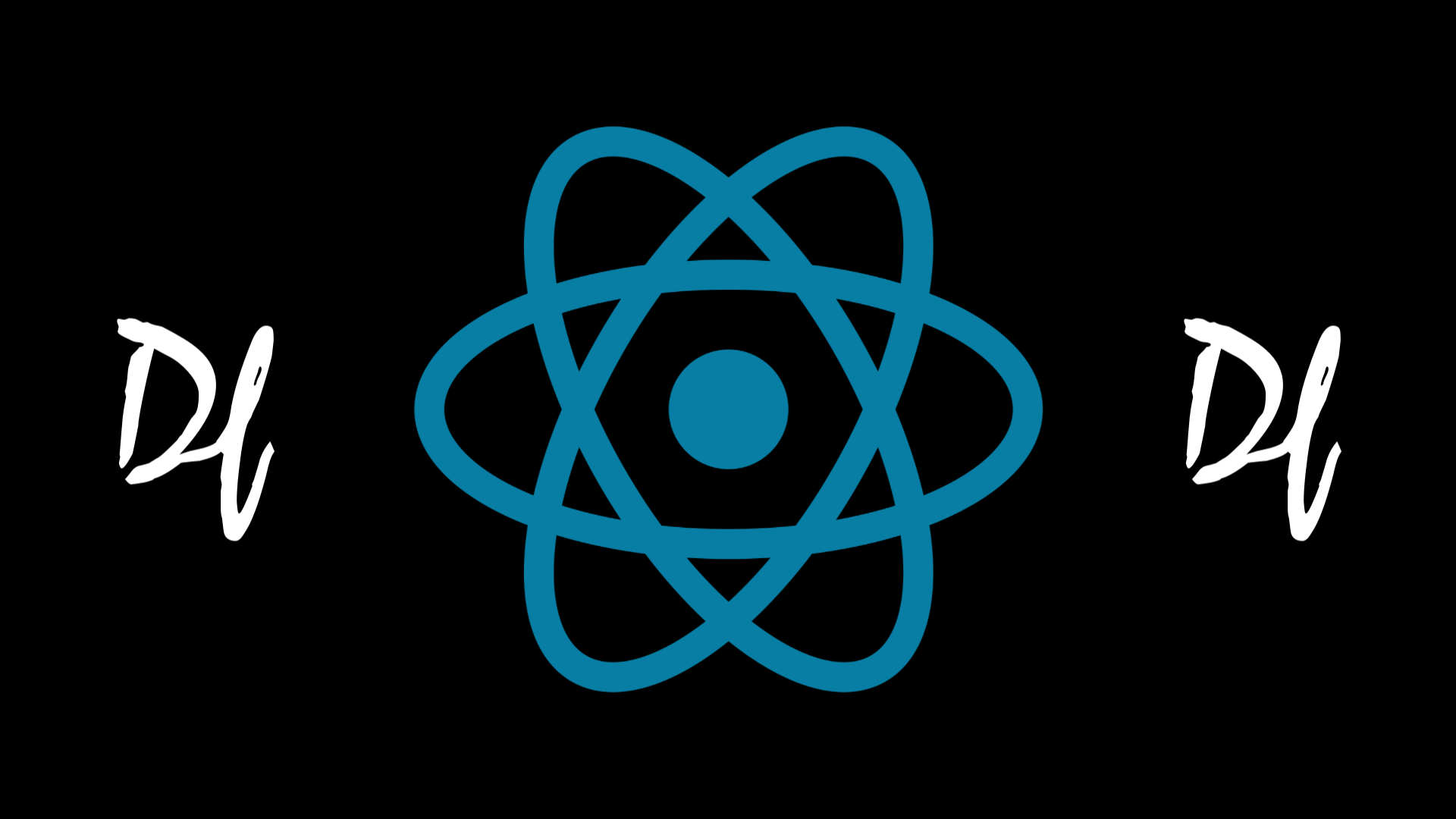
The recent update from the React Labs team, marks a pivotal moment in the evolution of React
The Dawn of the React Compiler
Perhaps the most groundbreaking announcement is the transition of the React Compiler from a research project to a core component powering Instagram.com in production. This leap is not just a technical achievement but a paradigm shift in optimizing React applications. The traditional reliance on manual memoization techniques like useMemo
, useCallback
, and memo
has been a necessary evil to prevent excessive re-renders. While effective, this approach introduced complexity and maintenance challenges. The introduction of the React Compiler signifies a move towards automatic optimization, ensuring UI components re-render only when necessary, without the developer's manual intervention.
React: Manual Memoization vs. React Compiler Optimization
Old Approach: Manual Memoization
Before the React Compiler, developers often used React.memo
, useMemo
, and useCallback
to avoid unnecessary re-renders.
The React Compiler's ability to navigate the dynamic nature of JavaScript and enforce the "rules of React" for optimization heralds a new era of efficiency and developer experience. The compiler can safely optimize applications by modeling both JavaScript's flexibility and React's principles, even when developers "bend the rules." This advancement not only enhances performance but also simplifies the development process, making React more approachable for new developers and more potent for veterans.
import React, { useState, useMemo } from 'react';
function ExpensiveComponent({ compute, value }) {
const result = useMemo(() => compute(value), [compute, value]);
return <div>Result: {result}</div>;
}
function App() {
const [count, setCount] = useState(0);
const square = useMemo(() => (x) => x * x, []);
return (
<div>
<ExpensiveComponent compute={square} value={count} />
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
New Approach: React Compiler Optimization
The React Compiler reduces the need for manual memoization as it optimizes re-renders automatically.
import React, { useState } from 'react';
function ExpensiveComponent({ compute, value }) {
// Assume the React Compiler automatically optimizes this component
const result = compute(value);
return <div>Result: {result}</div>;
}
function App() {
const [count, setCount] = useState(0);
const square = (x) => x * x;
return (
<div>
<ExpensiveComponent compute={square} value={count} />
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
In the new approach, the same performance benefits are expected without cluttering the code with memoization hooks, thanks to the React Compiler's automatic optimization.
React Actions vs. Vue and Angular for Data Handling
React with Actions
React's new Actions simplify data handling, especially for forms, making client-server communication more straightforward.
// React with Actions
function SearchForm() {
const search = async (formData) => {
// Perform the search operation
};
return (
<form action={search}>
<input name="query" />
<button type="submit">Search</button>
</form>
);
}
Vue: Method-based Data Handling
Vue handles form submissions and data handling through methods within the Vue instance.
<template>
<form @submit.prevent="search">
<input v-model="query" />
<button type="submit">Search</button>
</form>
</template>
<script>
export default {
data() {
return {
query: '',
};
},
methods: {
async search() {
// Perform the search operation
},
},
};
</script>
Angular: Reactive Forms
Angular often utilizes reactive forms for handling form submissions, offering a robust set of utilities for managing form state and validation.
import { Component } from '@angular/core';
import { FormControl } from '@angular/forms';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-search-form',
template: `<form (ngSubmit)="search()">
<input [formControl]="query" />
<button type="submit">Search</button>
</form>`,
})
export class SearchFormComponent {
query = new FormControl('');
constructor(private http: HttpClient) {}
search() {
// Perform the search operation
}
}
Comparison and Implications
React Compiler Optimization: This innovation significantly simplifies the developer experience in React by automating what used to be a manual and error-prone process. Compared to Vue and Angular, which are not directly equivalent to React's compiler-based optimization, React's approach can potentially lead to performance improvements without additional developer overhead.
React Actions: This feature simplifies handling form submissions and client-server data interactions, making React more comparable to Angular's reactive forms in handling form state and server communication. Vue's method-based approach, while straightforward, doesn't provide built-in state management for server interactions, unlike React Actions, which manage the lifecycle and state of the data submission.
Expanding the Horizon with Actions
Introducing Actions extends React's capabilities beyond the client side, offering a unified approach to handling data submissions and mutations across client and server environments. This feature simplifies form handling and server communication, making it more intuitive and efficient. The ability to manage asynchronous operations and optimistic UI updates seamlessly within React's ecosystem is a testament to the framework's commitment to developer efficiency and application performance.
Canary Releases: A Step Towards Open Innovation
The shift towards Canary releases represents a significant change in React's development philosophy. The React team embraces open innovation by involving the community in finalizing new features. This approach accelerates the development process and ensures that new features are battle-tested and refined based on community feedback before their stable release.
Looking Forward to React 19
The announcement of React 19 and its inclusion of all these advancements signifies a significant milestone. The focus on compatibility across different environments, alongside improvements like Asset Loading, Document Metadata, and support for Web Components, underscores React's commitment to providing a comprehensive development experience. React 19's emphasis on breaking changes for progress and improvement illustrates a forward-thinking mindset, ensuring React remains at the forefront of web development technologies.
Concluding Thoughts
The updates from React Labs mark a transformative period in the React ecosystem. The move towards automatic optimization with the React Compiler, the introduction of Actions for streamlined data handling, and the shift towards Canary releases for community-driven development all indicate a brighter, more efficient future for React developers. These advancements not only enhance the performance and usability of React applications but also reaffirm React's position as a leading framework in the ever-evolving landscape of web development.