Twelve-Factor App | Execute the App as Stateless Processes
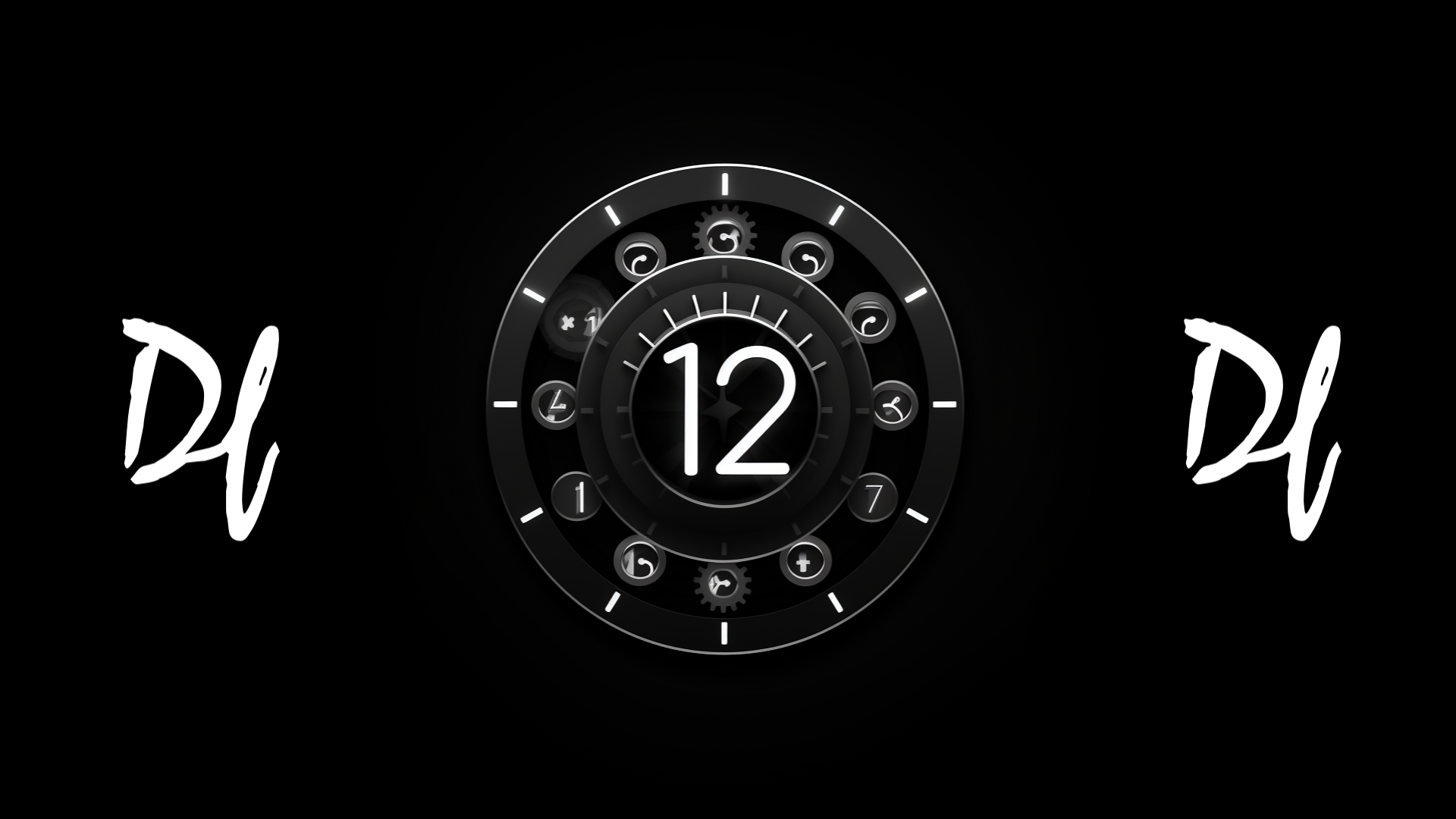
The sixth factor of the Twelve-Factor App methodology emphasizes executing the app as one or more stateless processes.
Why Execute as Stateless Processes?
Stateless processes are those that do not store any data related to the client session within the process itself. This approach offers several advantages:
Benefits:
- Scalability: Enables easy horizontal scaling.
- Reliability: Minimizes risks associated with process crashes.
- Maintainability: Simplifies the management and deployment of processes.
How to Execute as Stateless Processes
Avoid Local State
The state should not be stored in memory or the process's file system. Instead, it should be externalized.
Example: Using Redis for Session Management
import redis
from flask import Flask, session
app = Flask(__name__)
app.config['SESSION_TYPE'] = 'redis'
app.config['SESSION_PERMANENT'] = False
app.config['SESSION_USE_SIGNER'] = True
app.config['SESSION_KEY_PREFIX'] = 'myapp:'
app.config['SESSION_REDIS'] = redis.StrictRedis(host='redis', port=6379)
Utilize Stateless Protocols
HTTP, being a stateless protocol, aligns well with this approach.
Implement Horizontal Scaling
Stateless processes enable easy scaling by adding or removing instances.
Example: Kubernetes Deployment
apiVersion: apps/v1
kind: Deployment
metadata:
name: myapp
spec:
replicas: 3
...
Deployment Strategies with Stateless Processes
Managing stateless processes is crucial for seamless scaling and reliability.
Example:
- Build Stage: Ensure that the application is designed to be stateless.
- Deployment Stage: Implement horizontal scaling to handle varying loads.
- Monitoring Stage: Monitor process health and automatically replace failed instances.
Executing an application as stateless processes aligns with modern, cloud-native architecture principles.